在flink异步IO中通过lua脚本访问Redis,对MQ里无序的数据进行预排序合并。
一般是一条数据的不同状态顺序或增删改顺序,且乱序的延迟不大。
lua IDE
https://studio.zerobrane.com/
https://download.zerobrane.com/ZeroBraneStudioEduPack-1.80-macos.dmg
如果只使用redis.lua,可以把redis.lua放到lua path下面:
https://github.com/nrk/redis-lua/edit/version-2.0/src/redis.lua
http://luarocks.github.io/luarocks/releases/
http://luarocks.github.io/luarocks/releases/luarocks-3.1.3.tar.gz
https://github.com/luarocks/luarocks/wiki/Installation-instructions-for-macOS
安装lua5.3.5、lua-cjson和redis-lua
需要注意的是redis服务器端是支持cjson的,最好确认,还要注意具体语法是否支持。
1$ wget http://www.lua.org/ftp/lua-5.3.5.tar.gz
2$ tar xvf lua-5.3.5.tar.gz
3$ cd lua-5.3.5
4$ make macosx
5$ sudo make install
6$ lua -v
7Lua 5.3.5 Copyright (C) 1994-2018 Lua.org, PUC-Rio
8
9$ git clone https://github.com/mpx/lua-cjson.git
10$ cd lua-cjson
11$ vi Makefile
12CJSON_LDFLAGS = -bundle -undefined dynamic_lookup
13#FPCONV_OBJS = fpconv.o
14CJSON_CFLAGS += -DUSE_INTERNAL_FPCONV
15CJSON_CFLAGS += -DIEEE_BIG_ENDIAN
16CJSON_CFLAGS += -pthread -DMULTIPLE_THREADS
17
18$ make
19$ make install
20
21$ brew update
22$ brew install luarocks
23$ luarocks install redis-lua
24
25$ vi ~/.bash_profile
26export ZBS=/Applications/ZeroBraneStudio.app/Contents/ZeroBraneStudio
27export LUA_PATH="./?.lua;$ZBS/lualibs/?/?.lua;$ZBS/lualibs/?.lua"
28
29$ source ~/.bash_profile复制
ZeroBraneStudio使用cjson报错
1error loading module 'cjson' from file '/usr/local/lib/lua/5.3/cjson.so':
2 dlopen(/usr/local/lib/lua/5.3/cjson.so, 6): no suitable image found. Did find:
3 /usr/local/lib/lua/5.3/cjson.so: mach-o, but wrong architecture
4 /usr/local/lib/lua/5.3/cjson.so: mach-o, but wrong architecture复制
解决思路:修改配置cfg/user.lua然后重启
https://stackoverflow.com/questions/47320554/wrong-architecture-error-when-loading-lgi
1lua53 binary that comes with ZeroBrane Studio is i386 executable (32bit), but the library you are using is probably 64bit, hence the message about the wrong architecture.
复制
1$ vi /Applications/ZeroBraneStudio.app/Contents/ZeroBraneStudio/cfg/user.lua
2path.lua53 = '/usr/local/bin/lua'复制
重启ZeroBraneStudio即可
示例程序
注意Since Lua 5.2, loadstring has been replaced by load.
1local cjson = require("cjson")
2
3local sampleJson = [[{"age":"18","testArray":{"array":[8,9,11,14,25]},"blog":"https://icocoro.me"}]]
4local data = cjson.decode(sampleJson)
5print(data["age"])
6print(data["testArray"]["array"][1])复制
1local redis = require 'redis'
2local host = "127.0.0.1"
3local port = 6379
4
5-- 定义为global 否则redis.call无法调用
6client = redis.connect(host, port)
7
8local response = client:ping()
9print(("response: %s"):format(response))
10
11redis.call = function(cmd, ...)
12 return assert(load('return client:'.. string.lower(cmd) ..'(...)'))(...)
13end
14
15local hk = 'etlpre_test_p0'
16local isExists = client:exists(hk)
17local h = redis.call("exists", hk)
18print(h)
19
20-- 先判断key是否已经存在
21if not isExists then
22 print(package.path)
23end复制
需要注意的是json字符串在decode之后成为table,就不能再encode为json字符串了!!!
Please note that nested tables can require a lot of memory to encode.
所以,针对简单JSON,比如只有一层{},解析JSON,然后拼接成新的JOSN字符串输出。
1-- 旧的消息 来的晚
2local i = cjson.decode(info)
3-- newest是最新的消息 来的早
4local j = cjson.decode(newest)
5-- 最新的消息在等待旧的消息到来后重新合并
6-- 遍历当前info,如果info里的key不在newest,则添加到newest里面
7
8local tbl = {(string.gsub(newest, '}', ''))}
9
10for key, value in pairs(i) do
11 if j[key] == nil and value ~= nil then
12 if type(value) == 'number' then
13 table.insert( tbl, (',"%s":%d'):format(key,value))
14 elseif type(value) == 'boolean' then
15 table.insert( tbl, (',"%s":%s'):format(key,value))
16 else
17 table.insert( tbl, (',"%s":"%s"'):format(key,value))
18 end
19 end
20end
21table.insert( tbl, '}')
22
23print(table.concat(tbl))复制
演示图片
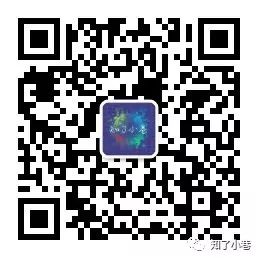
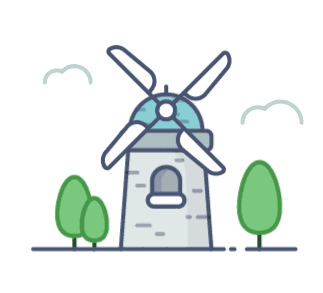
End
