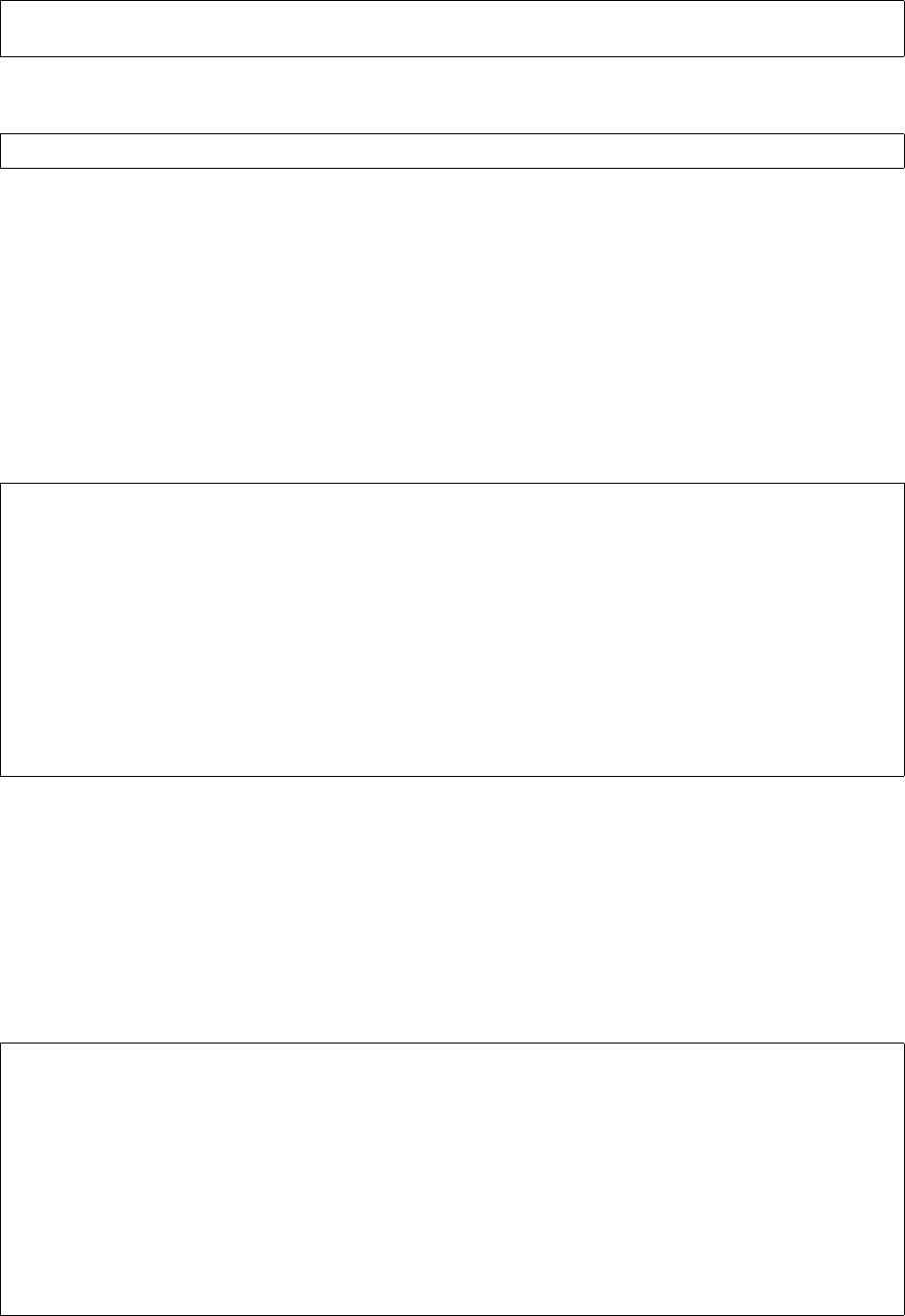
( )
with urllib.request.urlopen(req) as response:
the_page = response.read()
urllib.request Request FTP
req = urllib.request.Request('ftp://example.com/')
HTTP Request
HTTP
2.1
URL URL CGI web
HTTP POST HTML
POST POST
HTML data Request
urllib.parse
import urllib.parse
import urllib.request
url = 'http://www.someserver.com/cgi-bin/register.cgi'
values = {'name' : 'Michael Foord',
'location' : 'Northampton',
'language' : 'Python' }
data = urllib.parse.urlencode(values)
data = data.encode('ascii') # data should be bytes
req = urllib.request.Request(url, data)
with urllib.request.urlopen(req) as response:
the_page = response.read()
Note that other encodings are sometimes required (e.g. for file upload from HTML forms - see HTML Specification,
Form Submission for more details).
If you do not pass the data argument, urllib uses a GET request. One way in which GET and POST requests
differ is that POST requests often have ”side-effects”: they change the state of the system in some way (for example
by placing an order with the website for a hundredweight of tinned spam to be delivered to your door). Though
the HTTP standard makes it clear that POSTs are intended to always cause side-effects, and GET requests never to
cause side-effects, nothing prevents a GET request from having side-effects, nor a POST requests from having no
side-effects. Data can also be passed in an HTTP GET request by encoding it in the URL itself.
:
>>> import urllib.request
>>> import urllib.parse
>>> data = {}
>>> data['name'] = 'Somebody Here'
>>> data['location'] = 'Northampton'
>>> data['language'] = 'Python'
>>> url_values = urllib.parse.urlencode(data)
>>> print(url_values) # The order may differ from below.
name=Somebody+Here&language=Python&location=Northampton
>>> url = 'http://www.example.com/example.cgi'
>>> full_url = url + '?' + url_values
>>> data = urllib.request.urlopen(full_url)
Notice that the full URL is created by adding a ? to the URL, followed by the encoded values.
3
微信搜索公众号 "Python编程时光" ,回复 "pycharm" 获取免安装永久破解的专业版PyCharm
评论