简介
创建实验样本数据
/*
Source Server : 192.168.0.180
Source Server Type : MongoDB
Source Server Version : 40404
Source Host : 192.168.0.180:27017
Source Schema : hero
Target Server Type : MongoDB
Target Server Version : 40404
File Encoding : 65001
Date: 12/02/2022 20:44:36
*/
// ----------------------------
// Collection structure for hero
// ----------------------------
db.getCollection("hero").drop();
db.createCollection("hero");
// ----------------------------
// Documents of hero
// ----------------------------
db.getCollection("hero").insert([ {
_id: ObjectId("62079bf20f3100001e003b93"),
name: "张士诚",
gender: "男",
position: "霸主",
note: "被朱元璋打败",
age: 37
} ]);
db.getCollection("hero").insert([ {
_id: ObjectId("62079bf20f3100001e003b94"),
name: "陈友谅",
gender: "男",
position: "霸主",
note: "被朱元璋打败",
age: 32
} ]);
db.getCollection("hero").insert([ {
_id: ObjectId("62079bf20f3100001e003b95"),
name: "刘伯温",
gender: "男",
position: "谋士",
note: "给朱元璋打工的",
age: 26
} ]);
db.getCollection("hero").insert([ {
_id: ObjectId("62079bf20f3100001e003b96"),
name: "徐 达",
gender: "男",
position: "元帅",
note: "给朱元璋打工的",
age: 19
} ]);
db.getCollection("hero").insert([ {
_id: ObjectId("62079bf20f3100001e003b97"),
name: "胡惟庸",
gender: "男",
position: "宰相",
note: "给朱元璋打工的",
age: 35
} ]);
db.getCollection("hero").insert([ {
_id: ObjectId("62079bf20f3100001e003b98"),
name: "常遇春",
gender: "男",
position: "侦察旅旅长",
note: "给朱元璋打工的",
age: 40
} ]);
db.getCollection("hero").insert([ {
_id: ObjectId("62079bf20f3100001e003b99"),
name: "韩林儿",
gender: "男",
position: "小可爱",
note: "朱元璋前boss的儿子",
age: 29
} ]);
db.getCollection("hero").insert([ {
_id: ObjectId("62079f90aa95b252f944843d"),
name: "徐寿辉",
gender: "男",
position: "霸主",
note: "被陈友亮打败",
_class: "com.example.springboot_mongodb_demo.enity.Hero",
age: 51
} ]);
db.getCollection("hero").insert([ {
_id: ObjectId("6207a111caf8ce23735db5cc"),
name: "姚广孝",
gender: "男",
position: "谋士",
note: "给朱元璋和朱棣打工的",
age: NumberInt("57"),
_class: "com.example.springboot_mongodb_demo.enity.Hero"
} ]);
db.getCollection("hero").insert([ {
_id: ObjectId("6207a7310f3100001e003b9a"),
name: "大明孝慈高皇后马氏",
gender: "女",
position: "皇后",
note: "朱元璋的皇后",
age: 39
} ]);
复制
写法样例
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
复制
# 应用名称
spring:
data:
mongodb:
host: 192.168.0.180
port: 27017
database: hero
application:
name: springboot_mongodb_demo
# 应用服务 WEB 访问端口
server:
port: 8080
复制
package com.zuoyang.springboot_mongodb_demo.enity;
public class Hero {
private String id;
private String name;
private String gender;
private String position;
private String note;
private int age;
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public String getPosition() {
return position;
}
public void setPosition(String position) {
this.position = position;
}
public String getNote() {
return note;
}
public void setNote(String note) {
this.note = note;
}
@Override
public String toString() {
return "Hero{" +
"id='" + id + '\'' +
", name='" + name + '\'' +
", gender='" + gender + '\'' +
", position='" + position + '\'' +
", note='" + note + '\'' +
", age=" + age +
'}';
}
}
复制
查询集合中全部数据
db.hero.find()
复制

/**
* 查询集合中全部数据
* db.hero.find()
*/
@Test
void findHeroAll() {
List<Hero> list = mongoTemplate.findAll(Hero.class,"hero");
list.stream().forEach(System.out::println);
System.out.println("Hello MongoDB!");
}
复制
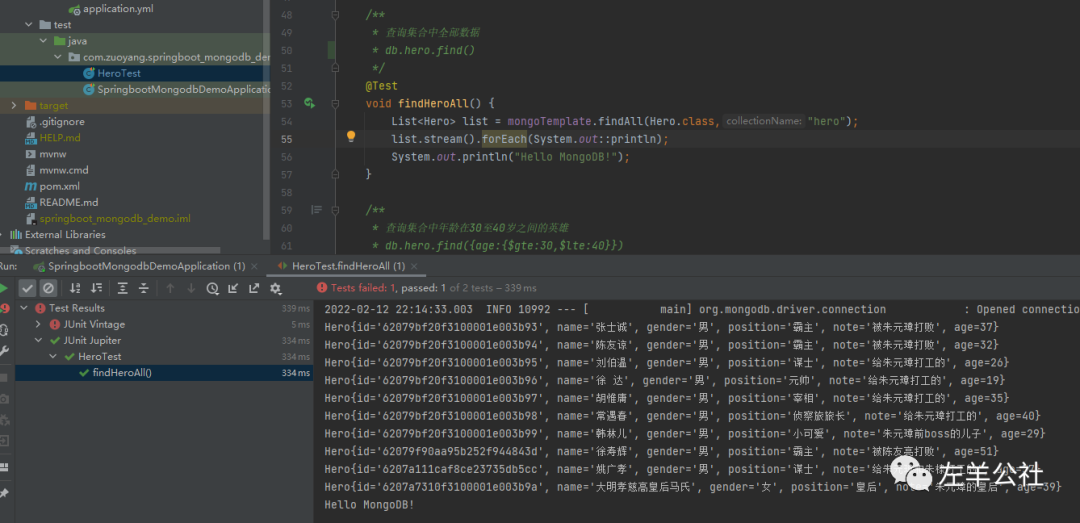
查询集合中年龄在30至40岁之间的英雄
db.hero.find({age:{$gte:30,$lte:40}})
复制

/**
* 查询集合中年龄在30至40岁之间的英雄
* db.hero.find({age:{$gte:30,$lte:40}})
*/
@Test
void findHerosByAge() {
Query query = new Query(Criteria.where("age").gte(30).lte(40));
List<Hero> list = mongoTemplate.find(query,Hero.class,"hero");
list.stream().forEach(System.out::println);
}
复制
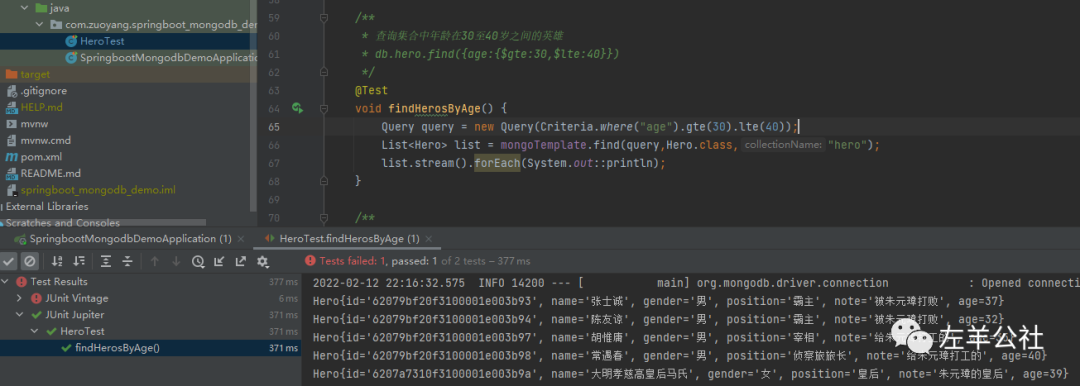
查询集合中年龄在30至40岁之间性别为女的英雄
db.hero.find({gender:"女",age:{$gte:30,$lte:40}})
复制

/**
* 查询集合中年龄在30至40岁之间性别为女的英雄
* db.hero.find({gender:"女",age:{$gte:30,$lte:40}})
*/
@Test
void findHerosByAgeAndGender() {
Query query = new Query(Criteria.where("age").gte(30).lte(40).and("gender").is("女"));
List<Hero> list = mongoTemplate.find(query,Hero.class,"hero");
list.stream().forEach(System.out::println);
}
复制
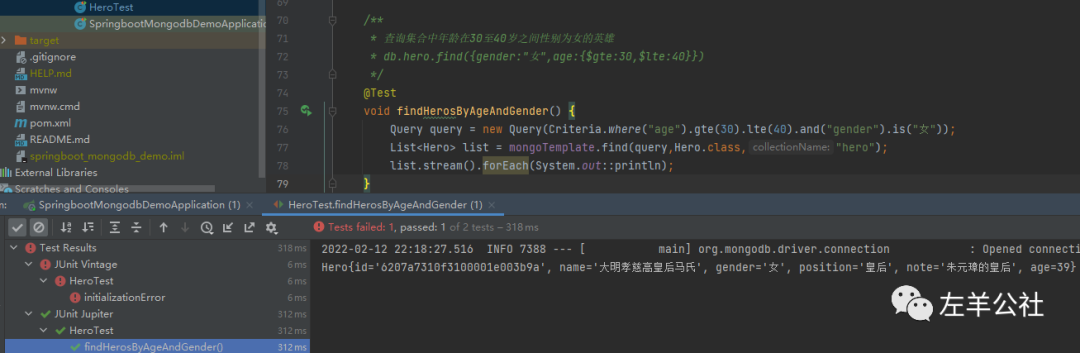
统计年龄在30岁到40岁性别为男英雄的年龄总和
db.hero.aggregate([
{$match:{gender:"男"}},
{$match:{age:{$gte: 30}}},
{$match:{age:{$lte: 40}}},
{$group:{_id:"$gender",total:{"$sum":"$age"}}}
])
复制
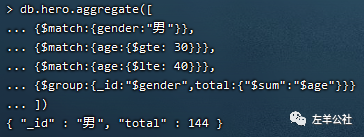
/**
* 统计年龄在30岁到40岁性别为男英雄的年龄总和
*
* db.hero.aggregate([
* {$match:{$gender:{$eq: "男"}}},
* {$match:{age:{$gte: 30}}},
* {$match:{age:{$lte: 40}}},
* {$group:{_id:"$gender",total:{"$sum":"$age"}}}
* ])
*/
@Test
void sumAgeByAge(){
List<AggregationOperation> aggregateOperations = new ArrayList<>();
// {$match:{$gender:{$eq: "男"}}}
aggregateOperations.add(Aggregation.match((Criteria.where("gender").is("男"))));
// {$match:{age:{$gte: 30}}}
aggregateOperations.add(Aggregation.match((Criteria.where("age").gte(30))));
// {$match:{age:{$lte: 40}}}
aggregateOperations.add(Aggregation.match((Criteria.where("age").lte(40))));
// {$group:{_id:"$gender",total:{"$sum":"$age"}}}
aggregateOperations.add(Aggregation.group("gender").sum("age").as("total"));
// 整合Mongo脚本
Aggregation aggregation = Aggregation.newAggregation(aggregateOperations);
// 执行Momgo脚本
AggregationResults<Map> aggregationResults = mongoTemplate.aggregate(aggregation,"hero", Map.class);
// 打印返回值
aggregationResults.getMappedResults().stream().forEach(System.out::println);
System.out.println((double) aggregationResults.getMappedResults().get(0).get("total"));
}
复制
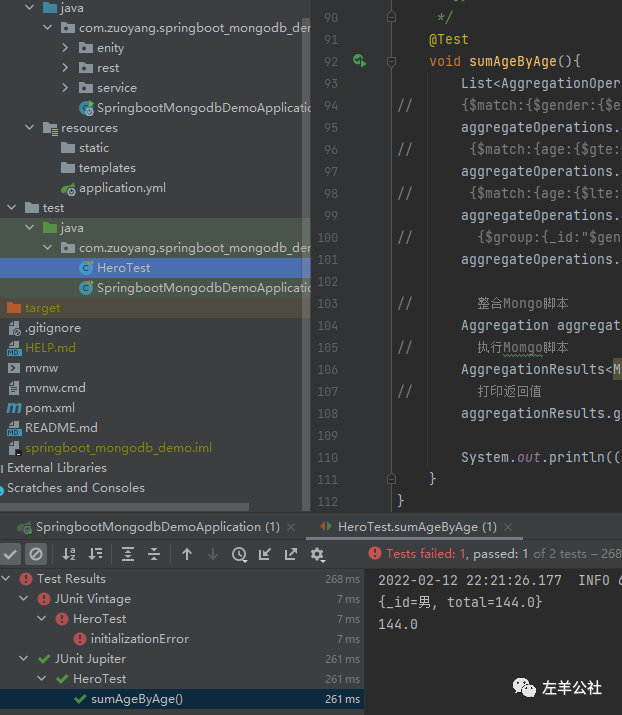
工程化
package com.zuoyang.springboot_mongodb_demo.service;
import com.zuoyang.springboot_mongodb_demo.enity.Hero;
import java.util.List;
public interface HeroService {
List<Hero> findHeroAll();
List<Hero> findHerosByAge();
List<Hero> findHerosByAgeAndGender();
double sumAgeByAge();
}
复制
package com.zuoyang.springboot_mongodb_demo.service.impl;
import com.zuoyang.springboot_mongodb_demo.enity.Hero;
import com.zuoyang.springboot_mongodb_demo.service.HeroService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.data.mongodb.core.aggregation.Aggregation;
import org.springframework.data.mongodb.core.aggregation.AggregationOperation;
import org.springframework.data.mongodb.core.aggregation.AggregationResults;
import org.springframework.data.mongodb.core.query.Criteria;
import org.springframework.data.mongodb.core.query.Query;
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
@Service
public class HeroServiceImpl implements HeroService {
@Autowired
private MongoTemplate mongoTemplate;
/**
* 查询集合中全部数据
*/
@Override
public List<Hero> findHeroAll() {
List<Hero> list = mongoTemplate.findAll(Hero.class,"hero");
list.stream().forEach(System.out::println);
System.out.println("Hello MongoDB!");
return list;
}
/**
* 查询集合中年龄在30至40岁之间的英雄
* db.hero.find({age:{$gte:30,$lte:40}})
*/
@Override
public List<Hero> findHerosByAge() {
Query query = new Query(Criteria.where("age").gte(30).lte(40));
List<Hero> list = mongoTemplate.find(query,Hero.class,"hero");
list.stream().forEach(System.out::println);
return list;
}
/**
* 查询集合中年龄在30至40岁之间性别为女的英雄
* db.hero.find({gender:"女",age:{$gte:30,$lte:40}})
*/
@Override
public List<Hero> findHerosByAgeAndGender() {
Query query = new Query(Criteria.where("age").gte(30).lte(40).and("gender").is("女"));
List<Hero> list = mongoTemplate.find(query,Hero.class,"hero");
list.stream().forEach(System.out::println);
return list;
}
/**
* 统计年龄在30岁到40岁性别为男英雄的年龄总和
*
* db.hero.aggregate([
* {$match:{$gender:{$eq: "男"}}},
* {$match:{age:{$gte: 30}}},
* {$match:{age:{$lte: 40}}},
* {$group:{_id:"$gender",total:{"$sum":"$age"}}}
* ])
*/
@Override
public double sumAgeByAge(){
List<AggregationOperation> aggregateOperations = new ArrayList<>();
// {$match:{$gender:{$eq: "男"}}}
aggregateOperations.add(Aggregation.match((Criteria.where("gender").is("男"))));
// {$match:{age:{$gte: 30}}}
aggregateOperations.add(Aggregation.match((Criteria.where("age").gte(30))));
// {$match:{age:{$lte: 40}}}
aggregateOperations.add(Aggregation.match((Criteria.where("age").lte(40))));
// {$group:{_id:"$gender",total:{"$sum":"$age"}}}
aggregateOperations.add(Aggregation.group("gender").sum("age").as("total"));
// 整合Mongo脚本
Aggregation aggregation = Aggregation.newAggregation(aggregateOperations);
// 执行Momgo脚本
AggregationResults<Map> aggregationResults = mongoTemplate.aggregate(aggregation,"hero", Map.class);
// 打印返回值
aggregationResults.getMappedResults().stream().forEach(System.out::println);
return Double.valueOf((Double) aggregationResults.getMappedResults().get(0).get("total"));
}
}
复制
package com.zuoyang.springboot_mongodb_demo.rest;
import com.zuoyang.springboot_mongodb_demo.enity.Hero;
import com.zuoyang.springboot_mongodb_demo.service.HeroService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
@RequestMapping("/hero")
public class HeroController {
@Autowired
private HeroService heroService;
/**
* 查询集合中年龄在30至40岁之间性别为女的英雄
*/
@GetMapping("/findHerosByAgeAndGender")
public List<Hero> findHerosByAgeAndGender() {
List<Hero> list = heroService.findHerosByAgeAndGender();
return list;
}
/**
* 查询集合中全部数据
*/
@GetMapping("/findHeroAll")
public List<Hero> findHeroAll() {
List<Hero> list = heroService.findHeroAll();
return list;
}
/**
* 查询集合中年龄在30至40岁之间的英雄
*/
@GetMapping("/findHerosByAge")
public List<Hero> findHerosByAge() {
List<Hero> list = heroService.findHerosByAge();
return list;
}
/**
* 统计年龄在30岁到40岁性别为男英雄的年龄总和
*/
@GetMapping("/sumAgeByAge")
public double sumAgeByAge(){
return heroService.sumAgeByAge();
}
}
复制
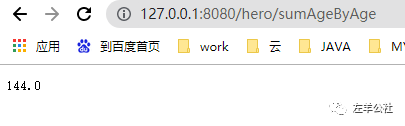
结语
[
END
]
文章转载自左羊公社,如果涉嫌侵权,请发送邮件至:contact@modb.pro进行举报,并提供相关证据,一经查实,墨天轮将立刻删除相关内容。
评论
相关阅读
【专家观点】罗敏:从理论到真实SQL,感受DeepSeek如何做性能优化
墨天轮编辑部
1269次阅读
2025-03-06 16:45:38
【专家有话说第五期】在不同年龄段,DBA应该怎样规划自己的职业发展?
墨天轮编辑部
1268次阅读
2025-03-13 11:40:53
2025年2月国产数据库大事记
墨天轮编辑部
992次阅读
2025-03-05 12:27:34
2025年2月国产数据库中标情况一览:GoldenDB 3500+万!达梦近千万!
通讯员
875次阅读
2025-03-06 11:40:20
2月“墨力原创作者计划”获奖名单公布
墨天轮编辑部
452次阅读
2025-03-13 14:38:19
AI的优化能力,取决于你问问题的能力!
潇湘秦
418次阅读
2025-03-11 11:18:22
优炫数据库成功应用于国家电投集团青海海南州新能源电厂!
优炫软件
339次阅读
2025-03-21 10:34:08
达梦数据与法本信息签署战略合作协议
达梦数据
291次阅读
2025-03-06 09:26:57
国产化+性能王炸!这套国产方案让 3.5T 数据 5 小时“无感搬家”
YMatrix
274次阅读
2025-03-13 09:51:26
磐维数据库对外门户全新升级!
磐维数据库
240次阅读
2025-03-04 15:32:59