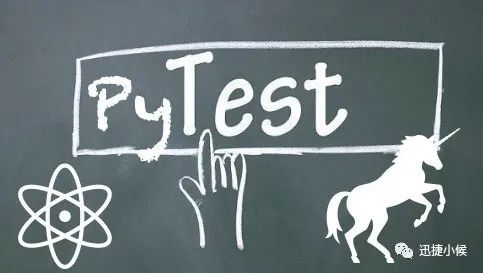
Pytest
在pytest中,如果想要把我们写好的测试用例进行分类的话,有什么办法可以实现呢?利用pytest.mark可以实现这一点。mark翻译过来就是标记的意思,它可以给我们的用例打上标记,这样我们就可以对用例进行分类。
01
pytest的mark机制
在pytest中,我们使用@pytest.mark.自定义变量即可标记测试用例。看下面的代码:
import pytest # 导入pytest
@pytest.mark.smoke # 标记用例为冒烟测试用例
def test_001():
print("test_001 done")
@pytest.mark.smoke # 标记用例为冒烟测试用例
def test_002():
print("test_002 done")
@pytest.mark.test # 标记用例为普通用例
def test_003():
print("test_003 done")
if __name__ == '__main__': # 定义主函数
pytest.main() # 调用pytest
复制
在上面我们对test_001和test_002两个测试函数标记了为smoke,也就是冒烟用例。对test_003标记为test,作为我们普通的用例。然后右键运行,发现如下结果:
============================= test session starts ==============================
platform darwin -- Python 3.8.2, pytest-6.2.1, py-1.10.0, pluggy-0.13.1 -- Users/zhangyingkai/PycharmProjects/pytest_demo/venv/bin/python
cachedir: .pytest_cache
rootdir: Users/zhangyingkai/PycharmProjects/pytest_demo
collecting ... collected 3 items
test_pytest.py::test_001 PASSED [ 33%]test_001 done
test_pytest.py::test_002 PASSED [ 66%]test_002 done
test_pytest.py::test_003 PASSED [100%]test_003 done
=============================== warnings summary ===============================
test_pytest.py:4
/Users/zhangyingkai/PycharmProjects/pytest_demo/test_pytest.py:4: PytestUnknownMarkWarning: Unknown pytest.mark.smoke - is this a typo? You can register custom marks to avoid this warning - for details, see https://docs.pytest.org/en/stable/mark.html
@pytest.mark.smoke # 标记用例为冒烟测试用例
test_pytest.py:9
/Users/zhangyingkai/PycharmProjects/pytest_demo/test_pytest.py:9: PytestUnknownMarkWarning: Unknown pytest.mark.smoke - is this a typo? You can register custom marks to avoid this warning - for details, see https://docs.pytest.org/en/stable/mark.html
@pytest.mark.smoke # 标记用例为冒烟测试用例
test_pytest.py:14
/Users/zhangyingkai/PycharmProjects/pytest_demo/test_pytest.py:14: PytestUnknownMarkWarning: Unknown pytest.mark.test - is this a typo? You can register custom marks to avoid this warning - for details, see https://docs.pytest.org/en/stable/mark.html
@pytest.mark.test # 标记用例为普通用例
-- Docs: https://docs.pytest.org/en/stable/warnings.html
======================== 3 passed, 3 warnings in 0.02s =========================
复制
在执行的结果中,我们可以发现,虽然测试用例执行成功了,但是也爆出了三个警告,为什么会有这些警告呢?我们看日志不难发现下面一段话:
PytestUnknownMarkWarning: Unknown pytest.mark.smoke - is this a typo? You can register custom marks to avoid this warning
复制
翻译过来大概就是:
PytestUnknownMark警告:未知pytest.mark.smoke-这是打字错误吗?您可以注册自定义标记以避免此警告
复制
可以看到 ,pytest这是让我们去注册一个什么玩意?自定义标记。那怎么注册自定义标记呢?我们需要在项目根目录下新建一个 pytest.ini的配置文件,内容如下:
[pytest]
markers=smoke
test
复制
这样的话,我们就可以把用户自定义的marker加进去了,我们使用命令行pytest --markers可以查看一下:
pytest --markers
@pytest.mark.smoke:
@pytest.mark.test:
@pytest.mark.filterwarnings(warning): add a warning filter to the given test. see https://docs.pytest.org/en/stable/warnings.html#pytest-mark-filterwarnings
@pytest.mark.skip(reason=None): skip the given test function with an optional reason. Example: skip(reason="no way of currently testing this") skips the test.
@pytest.mark.skipif(condition, ..., *, reason=...): skip the given test function if any of the conditions evaluate to True. Example: skipif(sys.platform == 'win32') skips the test if we are on the win32 platform. See https://docs.pytest.org/en/stable/reference.html#pytest-mark-skipif
@pytest.mark.xfail(condition, ..., *, reason=..., run=True, raises=None, strict=xfail_strict): mark the test function as an expected failure if any of the conditions evaluate to True. Optionally specify a reason for better reporting and run=False if you don't even want to execute the test function. If only specific exception(s) are expected, you can list them in raises, and if the test fails in other ways, it will be reported as a true failure. See https://docs.pytest.org/en/stable/reference.html#pytest-mark-xfail
@pytest.mark.parametrize(argnames, argvalues): call a test function multiple times passing in different arguments in turn. argvalues generally needs to be a list of values if argnames specifies only one name or a list of tuples of values if argnames specifies multiple names. Example: @parametrize('arg1', [1,2]) would lead to two calls of the decorated test function, one with arg1=1 and another with arg1=2.see https://docs.pytest.org/en/stable/parametrize.html for more info and examples.
@pytest.mark.usefixtures(fixturename1, fixturename2, ...): mark tests as needing all of the specified fixtures. see https://docs.pytest.org/en/stable/fixture.html#usefixtures
@pytest.mark.tryfirst: mark a hook implementation function such that the plugin machinery will try to call it first/as early as possible.
@pytest.mark.trylast: mark a hook implementation function such that the plugin machinery will try to call it last/as late as possible.
复制
有没有发现新大陆?pytest里面有很多自带的markers供用户使用,在最上面我们也可以看到自己的markers(smoke和test)。
那么现在再运行一下测试,就不会报警告了:
============================= test session starts ==============================
platform darwin -- Python 3.8.2, pytest-6.2.1, py-1.10.0, pluggy-0.13.1 -- Users/zhangyingkai/PycharmProjects/pytest_demo/venv/bin/python
cachedir: .pytest_cache
rootdir: Users/zhangyingkai/PycharmProjects/pytest_demo, configfile: pytest.ini
collecting ... collected 3 items
test_pytest.py::test_001 PASSED [ 33%]test_001 done
test_pytest.py::test_002 PASSED [ 66%]test_002 done
test_pytest.py::test_003 PASSED [100%]test_003 done
============================== 3 passed in 0.02s ===============================
复制
02
指定mark运行测试
我们如果想只运行冒烟测试用例,也就是被@pytest.mark.smoke标记的测试函数,怎么做呢?在pytest提供了-k参数,可以指定运行mark的用例:
pytest -k smoke
========================================================================================================================== test session starts ==========================================================================================================================
platform darwin -- Python 3.8.2, pytest-6.2.1, py-1.10.0, pluggy-0.13.1
rootdir: Users/zhangyingkai/PycharmProjects/pytest_demo, configfile: pytest.ini
collected 3 items 1 deselected / 2 selected
test_pytest.py .. [100%]
==================================================================================================================== 2 passed, 1 deselected in 0.03s ====================================================================================================================
复制
可以看到,这个时候只运行了两个测试用例,有一个测试用例被忽略了。我们也可以这样子运行不是smoke的测试用例:
pytest -k "not smoke"
========================================================================================================================== test session starts ==========================================================================================================================
platform darwin -- Python 3.8.2, pytest-6.2.1, py-1.10.0, pluggy-0.13.1
rootdir: /Users/zhangyingkai/PycharmProjects/pytest_demo, configfile: pytest.ini
collected 3 items / 2 deselected / 1 selected
test_pytest.py .
复制
使用起来还是很方便的!
那今天的内容到此为止啦,后续再给大家讲解更多的markers,对pytest进行更深入的学习。
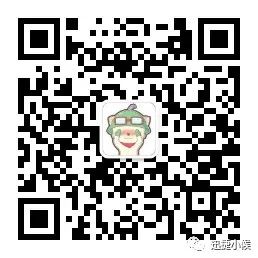
“A
Teemo