NO.1

面向对象与面向过程

面向对象程序设计(OOP):Object-Oriented Programming,面向过程程序设计(POP):Procedure-Oreinted Programming,面向对象和面向过程是两种不同的编程思想,理解两者的不同可以从下几个方面入手
一、表现形式:类库与函数库
在OOP中,封装在库中的是一个个类 在POP中,封装在库中的是一个个函数和变量
二、编程思想:对象与过程
有自己的属性,如荷载、尺寸
有自己的方法,如启动、行驶
NO.2

封装、继承与多态

"""封装(encapsulation):将同一类对象的共性抽象出来并集成在类中继承(inheritance):子类实例可以调用基类的任何属性和方法多态(polymorphism):继承于同一基类的不同派生类的实例对象的同一方法和属性可能是不同的"""# 我们以一个实例来理解这些概念# 下面的代码定义了一个People类,Student类是People的一个派生类# 定义了一个Person类,Child类是其派生类
复制
tips:左右滑动查看完整示例~
# <codecell> 封装 encapsulation# =============================================================================# 封装:将同一类对象的共性(属性和方法,或称之为数据和函数)抽象出来并集成在类中# =============================================================================import timeclass People(object): chinapopulation = 1.4e9 # 类属性,实例对象可以访问 __indiapopulation = 1.3e9 # 私有属性,子类无法继承基类的私有属性和方法 def sumpopulation(*country_population): # 类方法,实例对象不可调用 """ Parameters ---------- *country_population : tuple Population of imported countries. Returns ------- int Return to the total population of each country. """ return sum(country_population) def __init__(self, name, identity): # 构造方法,能且仅能在创建实例时自动调用一次 self.name = name self.identity = identity self.gender = 'male' if int(identity[-2]) % 2 else 'female' self.birth = '-'.join((identity[6:10], identity[10:12], identity[12:14])) self.age = ((time.time() - time.mktime( time.strptime(self.birth + ' 00:00:00', '%Y-%m-%d %H:%M:%S'))) // (60 * 60 * 24 * 365)) def myname(self): # 实例方法 """ Returns ------- name : string Return the name string. """ name = ''.join(('my name is \'', self.name, '\' !')) return name def mybirth(self): # self参数必须作为实例方法的第一个参数,用于传递实例名称 """ Returns ------- birth : string Calculates the date of birth using the id number and returns the date string. """ birth_year = self.identity[6:10] birth_month = self.identity[10:12] birth_day = self.identity[12:14] birth = '-'.join((birth_year, birth_month, birth_day)) return birth def mygender(self): """ Returns ------- gender : string Calculates the gender using the id number and returns a string. """ if int(self.identity[-2]) % 2: gender = 'male' else: gender = 'female' return gender def myage(self): """ Returns ------- age : float Calculates the age using the id number and returns a float. """ brith_datetime = self.birth + ' 00:00:00' # 出生时间均设为00:00:00 birth_strptime = time.strptime(brith_datetime, '%Y-%m-%d %H:%M:%S') birth_stamp = time.mktime(birth_strptime) age = (time.time() - birth_stamp) (60 * 60 * 24 * 365) return age# 注意:方法名和属性名称不能一样,否则调用方法时会出现类型异常# 注意:TypeError: 'str' object is not callableyi = People('yixunlian', '654321199812121234')# 查看属性yi.identity # 访问私有变量yi.nameyi.birthyi.genderyi.ageyi.chinapopulationyi._People__indiapopulation # 查看私有属性# 调用方法yi.myname()yi.mybirth()yi.mygender()yi.myage()# 类也是对象People.chinapopulationPeople._People__indiapopulation# <codecell> 继承 inheritance# =============================================================================# 继承:子类的实例对象可以调用基类的任何属性和方法!# =============================================================================class Student(People): def __init__(self, snumber, school, college, major, name, identity): People.__init__(self, name, identity) self.snumber = snumber self.school = school self.college = college self.major = major def info(self, city): infotuple = ('我叫', self.name, ',', '来自美丽的', city, ',', '今年', str(self.age)[:-2], '岁,现在于', self.school, self.college, '修读', self.major, '专业~') infostr = ''.join(infotuple) return infostryxl = Student('201612010007', '河海大学', '理学院', '应用统计', '小易', '500238199812121174')# 查看属性yxl.identityyxl.nameyxl.ageyxl.genderyxl.birthyxl.snumberyxl.schoolyxl.collegeyxl.major# 调用方法yxl.mygender()yxl.info('山城重庆')yxl.myage()yxl.mybirth()yxl.myname()yxl.chinapopulationyxl._Student__indiapopulation # AttributeError,基类私有属性无法继承!# <codecell> 多态 polymorphism# =============================================================================# 多态:继承于同一基类的不同派生类的实例对象的同一方法和属性可能是不同的!# =============================================================================class Person(object): def __init__(self, name, sex): self.name = name self.sex = sex def print_gender(self): if self.sex == "male": print("man") elif self.sex == "female": print("woman")class Child(Person): # Child继承Person,构造方法没有被重写则继承基类构造方法 def print_gender(self): # 基类方法被重写,子类同名方法将覆盖基类同名方法 if self.sex == "male": print("boy") elif self.sex == "female": print("girl")May = Child("May", "female")Peter = Person("Peter", "male") # 一个类被认为是其自身的派生类print(May.name, May.sex, Peter.name, Peter.sex)May.print_gender()Peter.print_gender()"""当子类和父类都存在相同的print_gender()方法时,子类的 print_gender()覆盖了父类的print_gender(),在代码运行时,会调用子类的 print_gender()这样,我们就获得了继承的另一个好处:多态。多态的好处就是,当我们需要传入更多的子类,例如新增 Teenagers、Grownups 等时,我们只需要继承 Person 类型就可以了,而print_gender()方法既可以直接不重写(即使用Person的),也可以重写一个特有的。这就是多态的意思。调用方只管调用,不管细节,而当我们新增一种Person的子类时,只要确保新方法编写正确,而不用管原来的代码。这就是著名的“开闭”原则:对扩展开放(Open for extension):允许子类重写方法函数对修改封闭(Closed for modification):不重写,直接继承父类方法函数"""
复制
NO.3

类与对象相关BIF

# =============================================================================# 相关BIF:Python提供了一些判断类间及类和对象关系的BIF,以及对对象属性的访问和修改# =============================================================================# issubclass(class, classinfo) 是否是子类?issubclass(Student, People)issubclass(Student, Student) # 一个类被认为是其自身的子类issubclass(Student, (People, str)) # classinfo可以是一个元组# isinstance(object, classinfo) object是否是classinfo或其子类的实例isinstance(1, int) # 1是整型实例isinstance(May, Person) # May是Person派生类Child的实例isinstance('hohai', (str, People)) # classinfo可以是一个元组# hasattr(object, attr) 测试object是否拥有属性attrhasattr(yi, 'age') # 注意属性名要用括号引起来,否则会抛出NameErrorhasattr(yi, 'myage') # 方法也是属性hasattr(yi, 'yourage')# getattr(object, attr[,default]) 获取object的属性,若不存在则返回默认值或抛出属性异常getattr(yi, 'age')getattr(yi, 'yourage')getattr(yi, 'yourage', '您访问的属性不存在')# setattr(object, attr, value) 修改或创建(若访问的不存在)属性值setattr(yi, 'age', 23)getattr(yi, 'age') # 成功将对象yi的age属性值修改为23setattr(yi, 'weight', 55)getattr(yi, 'weight') # 成功为对象yi添加属性weight并赋值55# delattr(object, attr) 删除object的属性,若属性不存在则抛出AttributeErrordelattr(yi, 'weight')delattr(yi, 'weight')
复制
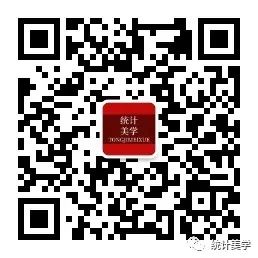
扫描二维码获取
更多精彩


统计美学