# 读入图像并绘制图像直方图
import matplotlib.pyplot as plt
import numpy as np
from PIL import Image #导入PIL库
import numpy as np
# 图像中引入中文字体
plt.rc("font",family='MicroSoft Yahei')
"""自己编写函数绘制直方图"""
def my_imhist(img):
"""
根据图像灰度值绘制直方图
Parameters
-----------
h:height of image
w:width of image
hist:用于储存统计图像每个像素点的灰度值个数
img:图像的灰度值
"""
# 获取图像的高与宽
h, w = img.shape
# 16位图像,拥有0-65535灰度值,首先各灰度频数都置为0
hist = [0] * 65536
#将图像第(i,j)个像素点对应的灰度值做计数储存在hist中
for i in range(h):
for j in range(w):
hist[img[i, j]] += 1
return hist
#读入图像
img = Image.open(r"axon01.tif")
#获取图像灰度值
# imgGray = img.convert('L')
img = np.asarray(img)
myhist=my_imhist(img)
plt.figure(figsize=(5,1))
plt.bar(range(65536), myhist, width=1)
plt.xlim(0,6000)
plt.title("自己编写my_imhist函数绘制的直方图")
"""调用函数hist绘制直方图"""
img = img.reshape(-1) #将图像展开成一个一维的numpy数组
plt.figure(figsize=(5,1))
plt.hist(img,128) #128
plt.title("调用hist绘制直方图")
plt.xlim(0,6000)
plt.show
# 读入图像并绘制图像直方图 import matplotlib.pyplot as plt import numpy as np from PIL import Image #导入PIL库 import numpy as np # 图像中引入中文字体 plt.rc("font",family='MicroSoft Yahei') """自己编写函数绘制直方图""" def my_imhist(img): """ 根据图像灰度值绘制直方图 Parameters ----------- h:height of image w:width of image hist:用于储存统计图像每个像素点的灰度值个数 img:图像的灰度值 """ # 获取图像的高与宽 h, w = img.shape # 16位图像,拥有0-65535灰度值,首先各灰度频数都置为0 hist = [0] * 65536 #将图像第(i,j)个像素点对应的灰度值做计数储存在hist中 for i in range(h): for j in range(w): hist[img[i, j]] += 1 return hist #读入图像 img = Image.open(r"axon01.tif") #获取图像灰度值 # imgGray = img.convert('L') img = np.asarray(img) myhist=my_imhist(img) plt.figure(figsize=(5,1)) plt.bar(range(65536), myhist, width=1) plt.xlim(0,6000) plt.title("自己编写my_imhist函数绘制的直方图") """调用函数hist绘制直方图""" img = img.reshape(-1) #将图像展开成一个一维的numpy数组 plt.figure(figsize=(5,1)) plt.hist(img,128) #128 plt.title("调用hist绘制直方图") plt.xlim(0,6000) plt.show
复制
# 读入图像并绘制图像直方图
import matplotlib.pyplot as plt
import numpy as np
from PIL import Image #导入PIL库
import numpy as np
# 图像中引入中文字体
plt.rc("font",family='MicroSoft Yahei')
"""自己编写函数绘制直方图"""
def my_imhist(img):
"""
根据图像灰度值绘制直方图
Parameters
-----------
h:height of image
w:width of image
hist:用于储存统计图像每个像素点的灰度值个数
img:图像的灰度值
"""
# 获取图像的高与宽
h, w = img.shape
# 16位图像,拥有0-65535灰度值,首先各灰度频数都置为0
hist = [0] * 65536
#将图像第(i,j)个像素点对应的灰度值做计数储存在hist中
for i in range(h):
for j in range(w):
hist[img[i, j]] += 1
return hist
#读入图像
img = Image.open(r"axon01.tif")
#获取图像灰度值
# imgGray = img.convert('L')
img = np.asarray(img)
myhist=my_imhist(img)
plt.figure(figsize=(5,1))
plt.bar(range(65536), myhist, width=1)
plt.xlim(0,6000)
plt.title("自己编写my_imhist函数绘制的直方图")
"""调用函数hist绘制直方图"""
img = img.reshape(-1) #将图像展开成一个一维的numpy数组
plt.figure(figsize=(5,1))
plt.hist(img,128) #128
plt.title("调用hist绘制直方图")
plt.xlim(0,6000)
plt.show
# 读入图像并绘制图像直方图 import matplotlib.pyplot as plt import numpy as np from PIL import Image #导入PIL库 import numpy as np # 图像中引入中文字体 plt.rc("font",family='MicroSoft Yahei') """自己编写函数绘制直方图""" def my_imhist(img): """ 根据图像灰度值绘制直方图 Parameters ----------- h:height of image w:width of image hist:用于储存统计图像每个像素点的灰度值个数 img:图像的灰度值 """ # 获取图像的高与宽 h, w = img.shape # 16位图像,拥有0-65535灰度值,首先各灰度频数都置为0 hist = [0] * 65536 #将图像第(i,j)个像素点对应的灰度值做计数储存在hist中 for i in range(h): for j in range(w): hist[img[i, j]] += 1 return hist #读入图像 img = Image.open(r"axon01.tif") #获取图像灰度值 # imgGray = img.convert('L') img = np.asarray(img) myhist=my_imhist(img) plt.figure(figsize=(5,1)) plt.bar(range(65536), myhist, width=1) plt.xlim(0,6000) plt.title("自己编写my_imhist函数绘制的直方图") """调用函数hist绘制直方图""" img = img.reshape(-1) #将图像展开成一个一维的numpy数组 plt.figure(figsize=(5,1)) plt.hist(img,128) #128 plt.title("调用hist绘制直方图") plt.xlim(0,6000) plt.show
复制
评论
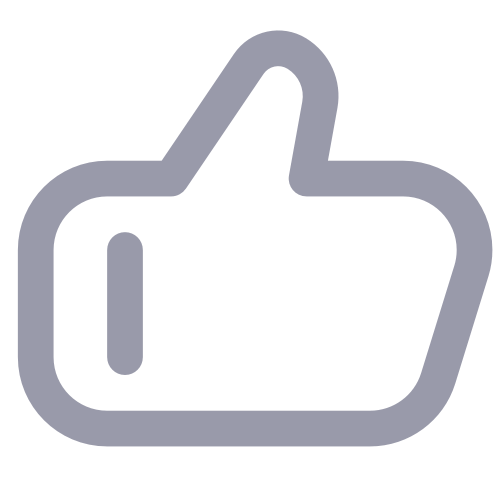